I've been wanting to play with 8-pin AVRs for awhile and finally got around to it.
Here's what's involved in downloading code to an ATtiny13, ATtiny85, and other AVR microprocessors. In this example, the ATtiny13 resides on a solderless breadboard for prototyping though you could simplify and (shameless promotion) buy my
ATtiny breakout boards. :)
Pinouts For In-System Programming
We'll use In-System Programming (ISP), a feature on all the AVR ATtiny and ATmega MCUs (as far as I know). It's a serial protocol, but unlike RS-232, there's a clock signal along with the TX and RX signals.
AVR's ISP cables have 6 pins: reset, clock (SCK), data to the chip (MOSI), data from the chip (MISO), as well as Vdd and GND. Follow the diagrams on my guide for
AVRISP Pinout for 8-pin, 14-pin, 20-pin ATtiny and ATmega328P.
AVR Programmers
You can use Pololu's inexpensive USB AVR programmer, PGM03A, as I did. It's a great programmer and I used the older version of it for a few years before upgrading. Plug the 6-pin ribbon cable into the programmer, then use jumper wires (I use these jumpers from hacktronics.com) to connect to the ATtiny on the breadboard. Add power (the newer PGM03A has 5V/GND pins) and you're ready to start programming. I've also used a JTAG ICE MkII which is expensive but has worked very well, too.
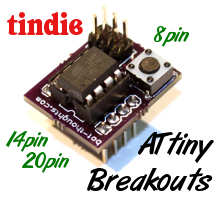
As mentioned (shameless promotion) my
eeZee Tiny includes AVRISP header, caps, reset switch and pullup. Add power and start programming.
Get the kit from my store on Tindie.
Choosing an IDE
I decided to try AVRstudio 4 for the first time and Pololu has
a tutorial on using AVRstudio with their programmer. It's easy to use an AVR programmer/debugger, as you might imagine. AVRstudio has nice debug features that accelerate troubleshooting in certain circumstances.
I'm currently using Eclipse with
AVR plug-in as my IDE as the editor is significantly better. To use the Pololu AVR Programmer, Configure plug-in that you're using an AVRISP Mk II on whatever serial port is allocated to the programmer.
Blink an LED on ATtiny
The embedded programming version of
hello world, as you probably know, is blinking an LED. We'll cast off the fluffy, protective blanket of Arduino and try this, AVR-style. It's not as easy, but it's not very hard either.
#include <avr io.h>
#include <avr interrupt.h>
#include <avr sleep.h>
#include <util delay.h>
/** ATtiny13 hello world; blink LED on Pin 2
*
* Michael Shimniok - http://www.bot-thoughts.com
*/
int main(int argc, char **argv)
{
DDRB |= _BV(3); // PB3 (pin2) as output
while (1) {
PORTB |= _BV(3); // turn on LED
_delay_ms(500);
PORTB &= ~_BV(3); // turn off LED
_delay_ms(500);
}
}
Additional Tips
To avoid programming glitches, keep the ATtiny13 clock speed up (9.6MHz, maybe even disable CLKDIV8) or reduce the programming speed.
The ATtiny13 is has very little flash or RAM but you'd be surprised at what you can do with it. It does have a timer with PWM modes and a multi-channel ADC. The form factor is, well, tiny, and the 8-pin AVRs are a great addition to the geek arsenal. I've been using 8-pin and 14-pin ATtinys quite a bit lately.
Did this help you? If so, do me a favor and share via redit, twitter, Google+, etc. Thanks!